Hey there, tech adventurers! Buckle up as we embark on a journey into the heart of Selenium WebDriver architecture. Today, we’re going to uncover the magical inner workings of this powerful tool that’s been driving testers and developers nuts… in a good way! So, grab your virtual magnifying glass, and let’s dive right in!
What’s Cooking in the Selenium Kitchen?
Picture this: You’re a master chef preparing a multi-course meal, and Selenium WebDriver is your trusty sous-chef. It’s the magic ingredient that helps you automate browser actions. But before you can taste success, let’s take a peek at what’s simmering beneath the Selenium kitchen’s hood.
WebDriver: Think of it as your digital chauffeur – it drives browsers like a pro, steering them through the treacherous roads of web pages. Whether you want to click buttons, fill out forms, or unleash other web interactions, WebDriver is your go-to sidekick. It’s like having a virtual butler that carries out your browsing commands without questioning your choices. “Yes, sir, right away, sir!”
Browser Drivers: These are the secret ingredients that make the whole dish work! Just like you need a specific key to unlock your treasure chest of chocolate (or whatever tickles your fancy), Selenium WebDriver relies on browser-specific drivers to establish a connection with the browsers. Each browser driver knows the ins and outs of its designated browser, so they can translate your high-level WebDriver commands into actions that browsers understand. It’s like having translators at a UN meeting – they ensure everyone speaks the same language.
JSON Wire Protocol: No, we’re not diving into a futuristic novel here. This is the language that WebDriver uses to communicate with browsers. It’s like the secret code between spies, ensuring that your commands are understood and executed by the browser correctly. Just think of it as the ultra-private language between best friends that no one else can crack – not even the nosy neighbors!
Execution Environment: Imagine your WebDriver script as a play, and the execution environment is the stage where it all comes to life. Your script writes the dialogues, but the execution environment provides the setting, props, and actors. This environment can be your local machine or even a remote server, depending on where you want the show to go on. It’s like having a theater that can be set up in your backyard or broadcast globally – the choice is yours!
Web Elements: These are the stars of the show – the elements on a web page that WebDriver can interact with. Buttons, text fields, checkboxes – they’re all part of the cast. You give WebDriver directions to find and manipulate these elements, just like a director instructs actors on stage. It’s like having your own army of tiny assistants who can fetch, click, and type for you!
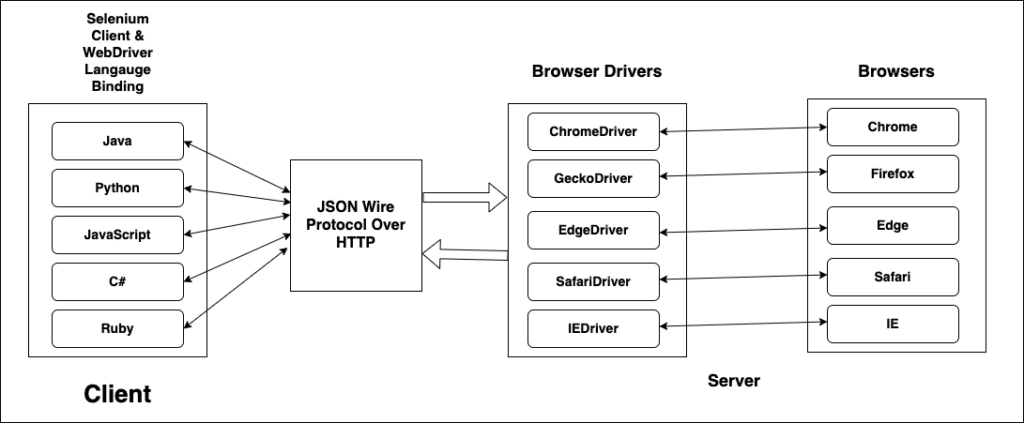
A Dash of Code: Sample Java and Selenium Recipe
Enough with the metaphors – let’s add a dash of code to this concoction! Here’s a tantalizing Java snippet that showcases how to set up WebDriver with Chrome:
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
public class SeleniumMagic {
public static void main(String[] args) {
// Set the path to ChromeDriver executable
System.setProperty("webdriver.chrome.driver", "path/to/chromedriver");
// Create a WebDriver instance for Chrome
WebDriver driver = new ChromeDriver();
// Navigate to a web page
driver.get("https://automationtestlab.com/");
// Perform your web automation wizardry here
// Close the browser
driver.quit();
}
}
Hold on to your chef’s hat – we’re about to decode this scrumptious script! Let’s break down the magic ingredients:
- Import Statements: These are like the chef’s secret ingredients list. They tell Java which classes you’ll be using from the Selenium library. It’s like preparing the spices before cooking up a storm.
- System.setProperty: Ever heard of a chef preheating the oven? This is the code’s way of setting the path to the ChromeDriver executable. It’s like telling your script where to find the oven.
- Creating WebDriver Instance: Here, you’re creating an instance of WebDriver specifically designed for Chrome. It’s like having a chauffeur ready to drive the Chrome browser wherever you want.
- Navigating to a Web Page: Just like a gourmet chef plates up the dish, this line tells WebDriver to navigate to the delicious web page at “https://automationtestlab.com/“.
- Web Automation Wizardry: Ah, the heart of the recipe! This is where you perform your automation magic – clicking buttons, filling out forms, and all that jazz. It’s like adding the secret sauce that makes the dish unforgettable.
- Closing the Browser: Every chef knows the importance of cleaning up after cooking. Here, you’re ensuring the browser window is closed properly when you’re done automating.
See that snippet? It’s like writing a magical spell – with a few lines of code, you summon WebDriver, tell it where to go, and let it work its charm. Voilà, your browser dances to your command!
Peeling Back the Layers: WebDriver Architecture
Imagine WebDriver as an onion – it has layers, and each layer plays a unique role in making your automation dreams come true.
- Your Test Script: This is where your journey begins. You write your test scripts in languages like Java, Python, or C#, making sure you’re spelling everything right – the browser won’t understand “clik” instead of “click”! Your script is like a script for a blockbuster movie – it guides the action and tells WebDriver what steps to perform.
- Client Libraries: These are like your friendly interpreters. They convert your high-level commands into the JSON Wire Protocol, which the browser can understand. With these libraries, you can interact with WebDriver without worrying about the nitty-gritty details. It’s like having a personal translator who ensures you never accidentally order a plate of snails when you actually want escargot!
- HTTP Server: WebDriver itself doesn’t directly communicate with browsers; it needs a translator. This server understands the JSON Wire Protocol and translates your commands into actions the browser can perform. Imagine this server as the ultimate concierge at a fancy hotel – you give it your commands, and it makes sure the browser receives them in the language it understands.
- Browser Drivers: Ah, the stars of our show! Each browser has its dedicated driver – ChromeDriver for Chrome, GeckoDriver for Firefox, and so on. These drivers act as intermediaries, interpreting your commands and translating them into browser-specific actions. Think of them as the interpreters at the United Nations of Browsers – they take your script and turn it into a language the browser can fluently speak.
- Browsers: Finally, we arrive at the browsers themselves. They receive the commands, perform the actions, and send back the results through the same pipeline. It’s like having a conversation with a web page – you speak WebDriver, it responds with the browser’s magic. This is where the curtains rise, and the real performance begins – the browser dances to the WebDriver’s tune!
- Web Element Identification: Just like a detective identifying a suspect, WebDriver needs to locate web elements accurately. It uses various strategies like ID, name, class, XPath, and CSS selectors to zero in on these elements. It’s like finding the right ingredient in your pantry – you know where it is, so you grab it without knocking over the spice rack!
Wrapping Up the Feast of Knowledge
And there you have it, folks! We’ve unveiled the layers of Selenium WebDriver architecture, explored the secret sauce that makes browser automation possible, and even spiced things up with some Java code. Now, you’re equipped to venture into the realm of automated testing with confidence. Remember, just like a master chef perfects their recipe over time, becoming a WebDriver whiz takes practice, patience, and a sprinkle of curiosity.
So, go forth and conquer the digital landscape, one automated click at a time! Happy testing, and may your code be bug-free and your browsers ever responsive. Until next time, stay curious and keep coding! 🚀
FAQs
Hold on to your hats – we’ve got some burning questions to address before we wrap up this journey!
Q1: What’s the deal with WebDriver and Selenium? Are they the same thing?
Great question! WebDriver is a part of the larger Selenium project. Think of Selenium as a toolset – it includes WebDriver for browser automation, but also Selenium IDE for recording tests, and Selenium Grid for distributing tests across different machines. WebDriver is the shining star of this toolkit, focusing solely on automating browsers. So, while WebDriver takes the spotlight, remember it’s just one star in the Selenium galaxy!
Q2: Can I automate any browser I want?
In theory, yes! But just like convincing a cat to take a bath, it’s easier said than done. WebDriver relies on browser drivers, and each browser has its quirks. ChromeDriver for Chrome, GeckoDriver for Firefox – you get the gist. So, while you can automate various browsers, you’ll need the right driver to make the magic happen. It’s like expecting a cat and a dog to understand each other without a common language – browsers need their translators (drivers)!
Q3: Is WebDriver a programming language?
Not quite. WebDriver is a library, not a programming language. It provides a set of functions and methods you can use in programming languages like Java, Python, and C#. These functions allow you to interact with browsers programmatically and automate your testing tasks. Think of WebDriver as a box of Lego pieces – you use them to build your automation structures using your favorite programming language as the blueprint!